Python Packages
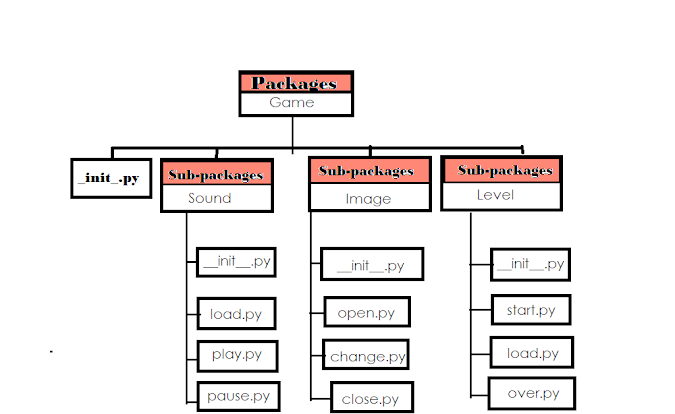
Python Packages Packages : Packages are a ways of structuring(organizing) Python's module namespace by using "dotted module names". A directory must contain a file named init.py in order for python to consider it as a package. This file can be left empty but we generally place the initialization code for that packages in this file. directory structure: Importing module from a packages We can import module from packages using the dot ( . ) operator. #import Game.Image.open Packages in Python, is basically group of modules.