Python3 for Loop
Python For Loop
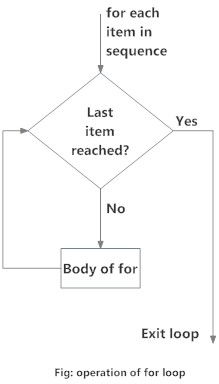
output:: 0
for Loop:
The for loop is used to iterate over a sequence (list, tuple, string) or other iterable objects.
Iterating over a sequence is called traversal.
Syntax:
for element in sequence:body of for
Here, elements is the variable that takes the value of the item inside the sequence on each iteration.
Loop continues until we reach the last item in the sequence.
Flow chart:
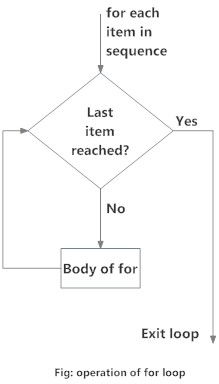
Eg:
lst=[10,20,30,40,50]product=1for ele in lst:product*=eleprint("product is: {}".format(product))
output:: product is: 12000000
for more info :
range() function :
We can generate a sequence of numbers using range() function. range(10) will generate numbers from 0 to 9(10 numbers).
Eg:
for i in range(10):print(i)
1
23456789
Eg:
for i in range(1,16,2):print(i)
output:: 1
3
5
7
9
11
13
15
Eg:
lst=["Ram", "Bharath", "Krishna", "Lakshman", "dharma raj"]for index in range(len(lst)):print(lst[index])
output:: Ram
Bharath
Krishna
Lakshman
dharma raj
for loop with else:
A for loop can have an optional else block as well. The else part is executed if the items in the sequence used in for loop exhausts.
* break statement can be used to stop a for loop, in such case, the else part is ignored.
Hence, a for loop's else part runs if no break occur.
Eg:
nums=[1,3,5]for item in nums:print(item)else:print("no item left in the list")
output: 1
3
5
no item left in the list
Eg:
nums=[1,2,3]for item in nums:print(item)if item%2==0:breakelse:print("no item left in the list")
output:: 1
2
Python program to display all prime numbers within an interval:
i=10j=50print("prime numbers between {} and {} are: ".format(i,j))for num in range(i,j+1):if num>1:isdivisible=False;for index in range(2,num):if num%index==0:isdivisible=True;if not isdivisible:print(num);
output:: prime numbers between 10 and 50 are:
11
13
17
19
23
29
31
37
41
43
47
Comments
Post a Comment