Python3 break and continue Statements
Python break and continue Statements
Python program to check given number is prime number or not (using break):
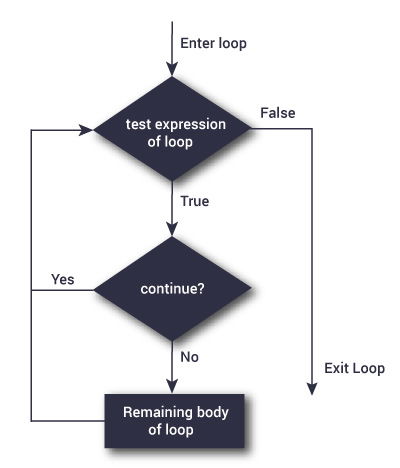
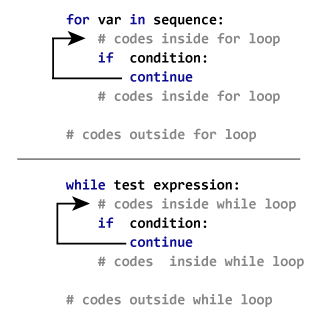
break and continue statements
In Python , break and continue statements can alter the flow of normal loop.
Loop iterate over a block of code until test expression is false, but sometimes we wish to terminate the current iteration or even the whole loop without checking test expression.
The break and continue statements are used in these cases.
Python Break Statement
Syntax:
break
Flow Chart:
Working:
Eg:
nums=[1,2,3,4]for num in nums:if num==4:breakprint(num)else:print("in the else block")print("outside of for loop")
Output:: 1
2
3
outside of for loop
num=int(input("enter a number: ")
isdivisible=False;
i=2;
while i<num:
if num % i==0:
isdivisible=True;
print("{} is divisible by {}".format(num,i))
break;
i+=1;
if isdivisible:
print("{} is not a prime number".format(num))
else:print("{} is a prime number".format(num))
output:: enter a number: 16
16 is divisible by 2
16 is not a prime number
Python Continue Statement
Syntax:
continue
Flow Chart:
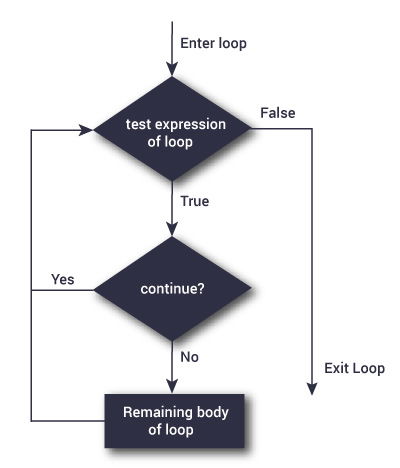
Working:
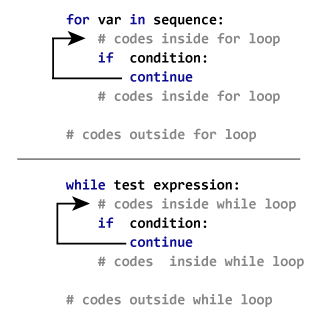
Eg:
nums=[1,2,3,4,5]for num in nums:if num%2==0:continueprint(num)else:print("else block")
output:: 1
3
5
else block
Comments
Post a Comment