Python3 if..... else
Python if .... else Statement
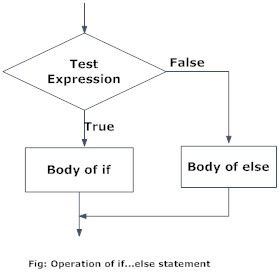
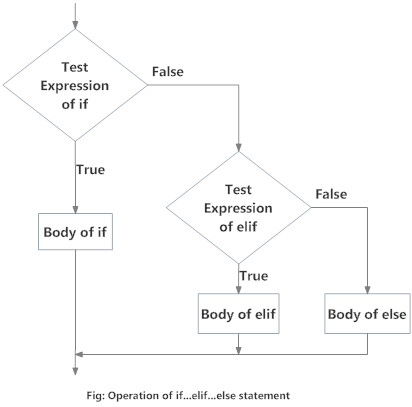
IF... ELSE STATEMENT :
The if... elif.. else statements is used in Python for decision making.
if statement syntax:
if test expression:
statement(s)
Flow chart :
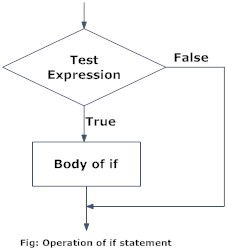
* The program evaluates the test expression and execute the statement(s) only if the test expression is true.
* If the test expression is false, the statements is not executed.
Eg:
num=25
if num=24:
print("The number is divisible by 2,3,6.")
print(" This will print always.")
output: This will print always.
IF.... ELSE STATEMENT :
Syntax::
if test expression:
body of if
else:
body of else
Flow chart :
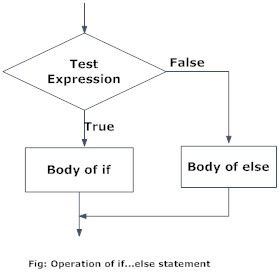
Eg:
num=4
if num>0:
print("Positive number")
else:
print("Negative number")
output: Positive number
IF...ELIF...ELSE STATEMENT :
Syntax::
if test expression:body of ifelif test expression:body of elifelse:body of else
Flow chart :
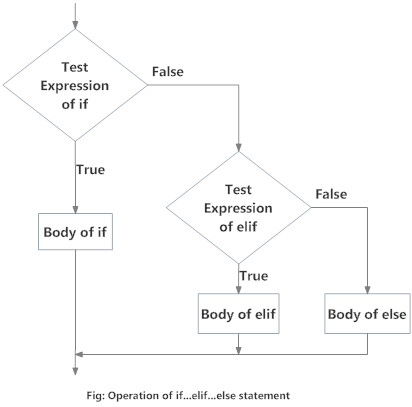
Eg:
num=0if num>0:print("Positive number")elif num==0:print("Zero")else:print("Negative number")
output:: Zero
NESTED IF STATEMENTS :
We can have a if.. elif..else statements inside another if.. elif... else statements. This is called nesting in computer programming.
Eg:
num=1if num>=0:if num==0:print("zero")else:print("positive number")else:print("negative number")
output: positive number
Python program to find the largest element among three numbers:
a=0;b=-2;c=7if a>b & a>c:print("The greater number is a ",a)elif b>c:print("The greater number is b ",b)else:print("The greater number is c ",c)
output:: The greater number is c 7
(or)a=6b=8c=3if (a>b) and (a>c):large=aelif (b>c):large=belse:large=cprint("The larger number is : {}".format(large))
output: The larger number is : 8
Comments
Post a Comment